Material Container Widget Tutorial
A container first surrounds the child with padding and then applies additional constraints to the padded extent. The container is then surrounded by additional empty space described from the margin.
During painting, the container first applies the given transform, then paints the decoration to fill the padded extent, then it paints the child, and finally paints the foregroundDecoration, also filling the padded extent.
Containers with no children try to be as big as possible unless the incoming constraints are unbounded, in which case they try to be as small as possible. Containers with children size themselves to their children. The width, height, and constraints arguments to the constructor override this.
Material Container Widget useful inputs
-
color: The color to paint the material. The Colors enum will apply here.
-
padding: Empty space to inscribe inside the decoration. The child, if any, is placed inside this padding. It describes by EdgeInsets
-
margin: Empty space to surround the decoration and child. It describes by EdgeInsets
-
alignment: Align the child within the container using Alignment Enum.
-
child: Take Widget class
-
decoration: The decoration to paint behind the child.
-
height: height of the widget, it’s take double value
-
width: width of the widget, it’s take double value
Material Container Widget example
Container(
child: Text(
"This is Container widget example",
),
color: Colors.black,
padding: EdgeInsets.all(50.0),
margin: EdgeInsets.all(50.0),
alignment: Alignment.center,
)
Full codes example
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main() => (runApp(ContainerExample()));
class ContainerExample extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Container Example Code'),
),
body: Container(
child: Text(
"This is Container widget example",
style: TextStyle(
color: Colors.green,
fontSize: 25,
),
),
color: Colors.black,
padding: EdgeInsets.all(50.0),
margin: EdgeInsets.all(50.0),
alignment: Alignment.center,
),
),
);
}
}
Output
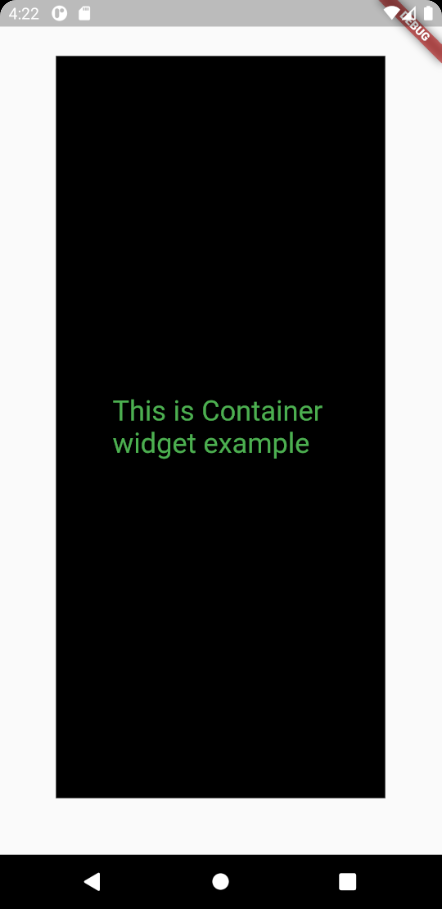
Container codes with decoration example
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main() => (runApp(ContainerExampleWithDecoration()));
class ContainerExampleWithDecoration extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Container Example Code'),
),
body: Container(
child: Text(
"This is Container widget example",
style: TextStyle(
color: Colors.red,
fontSize: 25,
),
),
decoration:
BoxDecoration(shape: BoxShape.circle, color: Colors.green),
padding: EdgeInsets.all(50.0),
margin: EdgeInsets.all(50.0),
alignment: Alignment.center,
),
),
);
}
}