CSS Box Model
The CSS Box Model is a fundamental concept that defines the layout and structure of HTML elements on a webpage. It comprises several components that outline the space and boundaries around an element.
Components of the Box Model
-
Margin : The space outside the border, defining the element’s space relative to other elements.
-
Border : Surrounds the padding and content, creating a visible or invisible boundary. It can have a specified width, style, and color.
-
Padding : Space between the content and the element’s border. It provides internal space within the element.
-
Content : The actual content area where text, images, or other media are displayed.
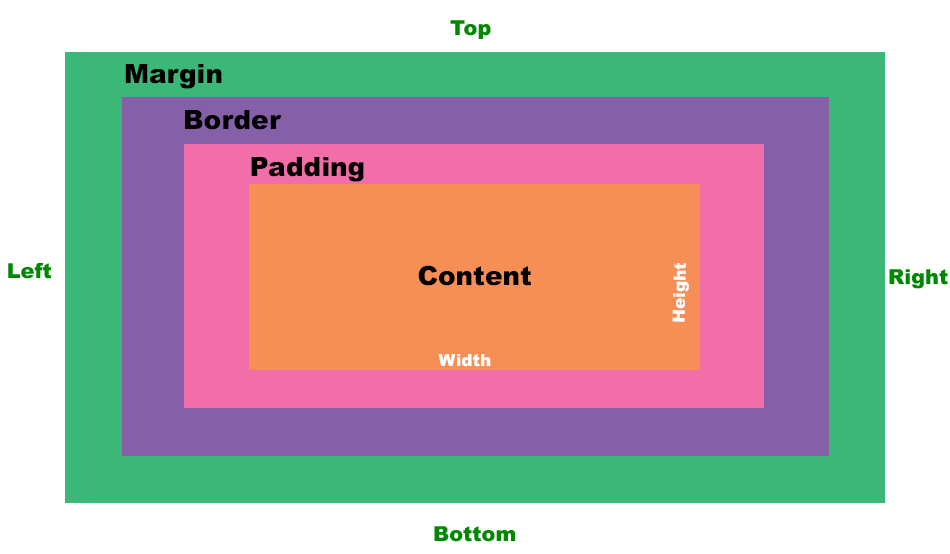
CSS Margin
The margin property is used to create space around an element, outside its border. It controls the distance between the element’s border and adjacent elements on a webpage.
Example
/* Shorthand - All sides with same value */
.element {
margin: 20px;
}
/* Shorthand - Vertical & Horizontal Margin */
.another-element {
margin: 10px 20px; /* top/bottom: 10px, left/right: 20px */
}
/* Shorthand - top, right, bottom, left */
.shorthand-full {
margin: 10px 20px 15px 5px; /* top: 10px, right: 20px, bottom: 15px, left:5px */
}
/* Individual Sides */
.one-more-element {
margin-top: 15px;
margin-right: 25px;
margin-bottom: 15px;
margin-left: 25px;
}
-
Shorthand : Sets the margin for all four sides of the element in one declaration.
-
Syntax :
margin: top right bottom left;
-
-
Individual Sides : Sets margin for specific sides of the element separately.
-
Syntax :
margin-top, margin-right, margin-bottom, margin-left
-
Units for Margin
-
Length Values : px, em, rem, %, etc.
-
auto : Allows the browser to calculate the margin.
-
CSS Border
The border property is used to define the border around an element. It allows you to specify the width, style, and color of the border.
Example
/* Shorthand - Sets all border properties */
.element {
border: 2px solid #000; /* width: 2px, style: solid, color: #000 (black) */
}
/* Individual Properties */
.another-element {
border-width: 1px;
border-style: dashed;
border-color: red;
}
-
Shorthand : Sets all the individual border properties (width, style, and color) in one declaration.
-
Syntax :
border: width style color;
-
-
Individual Properties : Sets border properties separately.
-
Syntax :
border-width, border-style, border-color
-
Border Components
-
border-width : Sets the width of the border.
-
Values : thin, medium, thick, or specific length values like 1px, 2px.
-
-
border-style : Sets the style of the border
-
Values : solid, dotted, dashed, double, groove, ridge, inset, outset, etc.
-
-
border-color : Sets the color of the border.
-
Values : Color names (e.g., red, blue), hexadecimal (#ff0000), RGB (rgb(255, 0, 0)), etc.
-
CSS Padding
The padding property is used to create space around the content inside an element, within its border. It controls the amount of space between the content and the element’s border.
Example
/* Shorthand - All sides with same value */
.element {
padding: 20px;
}
/* Shorthand - Vertical & Horizontal Padding */
.another-element {
padding: 10px 20px; /* top/bottom: 10px, left/right: 20px */
}
/* Shorthand - top, right, bottom, left */
.shorthand-full {
padding: 10px 20px 15px 5px; /* top: 10px, right: 20px, bottom: 15px, left:5px */
}
/* Individual Sides */
.one-more-element {
padding-top: 15px;
padding-right: 25px;
padding-bottom: 15px;
padding-left: 25px;
}
-
Shorthand : Sets the padding for all four sides of the element in one declaration.
-
Syntax :
padding: top right bottom left;
-
-
Individual Sides : Sets padding for specific sides of the element separately.
-
Syntax :
padding-top, padding-right, padding-bottom, padding-left
-
Units for Padding
-
Length Values : px, em, rem, %, etc.
-
auto : Allows the browser to calculate the padding.
-
CSS Box Model Content
In the CSS Box Model, the content area is the innermost part of an HTML element where the actual content, such as text, images, or other media, is displayed.
Example
.box {
width: 300px; /* Width defining the content area */
height: 200px; /* Height defining the content area */
padding: 20px; /* Padding around the content */
border: 2px solid #000; /* Border surrounding the content */
margin: 30px; /* Margin outside the border */
box-sizing: border-box; /* Including padding and border within the defined width and height */
}
Content Area Size
The size of the content area within an element is primarily controlled by the width and height properties. These properties determine the dimensions of the space where the actual content, such as text, images, or other media, is displayed.
-
width : Specifies the width of the content area.
-
Values : Length values (px, em, rem, %), auto, etc.
-
-
height : Determines the height of the content area.
-
Values : Length values (px, em, rem, %), auto, etc.
-
Content Box Sizing
The box-sizing property in CSS determines how the total width and height of an element are calculated, specifically regarding the content, padding, and border.
Example
.content-box {
box-sizing: content-box;
}
.border-box {
box-sizing: border-box;
}
content-box (default)
Default behavior where the width and height properties only apply to the content area. Padding and border are added to the specified width and height, increasing the total rendered size of the element.
-
Actual Width of an element : Width + Padding + Border
-
Actual Height of an element : Height + Padding + Border
border-box
Includes padding and border within the specified width and height. The content area adapts to the available space after accounting for padding and border dimensions.